- What is DevOps?
- DevOps is a set of practices that combines software development (Dev) and IT operations (Ops) to shorten the development lifecycle and provide continuous delivery with high software quality.
- What are the key components of DevOps?
- Continuous Integration (CI), Continuous Delivery (CD), Infrastructure as Code (IaC), Monitoring and Logging, Collaboration, and Automation.
- Explain Continuous Integration (CI).
- CI is a practice where developers frequently integrate code into a shared repository, usually several times a day. Each integration is verified by an automated build and automated tests.
- What is Continuous Delivery (CD)?
- CD is the practice of keeping the codebase deployable at any point and automating the deployment process, ensuring that code changes can be released to production quickly and safely.
- What are some popular CI/CD tools?
- Jenkins, GitLab CI, Travis CI, CircleCI, and Bamboo.
- What is Version Control?
- Version control is a system that records changes to a file or set of files over time so that you can recall specific versions later.
- What is Git?
- Git is a distributed version control system used to track changes in source code during software development.
- Explain the difference between Git and GitHub.
- Git is a version control system, while GitHub is a web-based platform that uses Git for version control and offers additional features like issue tracking, project management, and collaboration tools.
- How do you create a new branch in Git?
git branch <branch-name>
- How do you merge branches in Git?
git merge <branch-name>
- What is Docker?
- Docker is a platform for developing, shipping, and running applications in containers, which are lightweight, portable, and self-sufficient.
- What is a Docker image?
- A Docker image is a read-only template used to create Docker containers. It includes the application and all dependencies required to run it.
- What is a Docker container?
- A Docker container is a lightweight, portable, and self-sufficient package that includes the application code and its dependencies.
- How do you create a Docker image?
- By writing a
Dockerfile
and using the commanddocker build -t <image-name> .
- By writing a
- How do you run a Docker container?
docker run <image-name>
- What is Infrastructure as Code (IaC)?
- IaC is the practice of managing and provisioning computing infrastructure through machine-readable scripts, rather than through physical hardware configuration or interactive configuration tools.
- What are some popular IaC tools?
- Terraform, AWS CloudFormation, Ansible, and Puppet.
- Explain the difference between Terraform and Ansible.
- Terraform is a tool for building, changing, and versioning infrastructure using declarative configuration files, while Ansible is a configuration management tool that automates software provisioning, configuration management, and application deployment.
- How do you initialize a Terraform project?
terraform init
- How do you apply a Terraform configuration?
terraform apply
- Why is monitoring important in DevOps?
- Monitoring helps ensure system reliability, performance, and availability. It allows teams to detect issues early, understand system behavior, and make data-driven decisions.
- What are some popular monitoring tools?
- Prometheus, Grafana, Nagios, Zabbix, and Datadog.
- What is logging in the context of DevOps?
- Logging involves capturing, storing, and analyzing log data generated by applications and infrastructure to help diagnose and troubleshoot issues.
- What are some popular logging tools?
- ELK Stack (Elasticsearch, Logstash, Kibana), Splunk, Fluentd, and Graylog.
- What is the ELK Stack?
- The ELK Stack is a collection of three open-source products: Elasticsearch (search and analytics engine), Logstash (log pipeline tool), and Kibana (data visualization).
- What is Configuration Management?
- Configuration Management involves managing and maintaining the consistency of a product’s performance and its functional and physical attributes with its requirements, design, and operational information throughout its life.
- What are some popular Configuration Management tools?
- Ansible, Puppet, Chef, and SaltStack.
- Explain the role of Ansible in Configuration Management.
- Ansible automates software provisioning, configuration management, and application deployment. It uses simple, human-readable YAML templates.
- How do you define a playbook in Ansible?
- An Ansible playbook is defined using YAML format and describes the tasks to be executed on a set of hosts.
- What is a Puppet manifest?
- A Puppet manifest is a file containing Puppet code, written in the Puppet language, that describes how resources should be configured on a system.
- What is a Cloud Platform?
- A cloud platform is a suite of services and tools provided by a cloud provider (such as AWS, Azure, GCP) to build, deploy, and manage applications and infrastructure.
- What are the key services provided by AWS?
- Compute (EC2), Storage (S3), Database (RDS), Networking (VPC), IAM (Identity and Access Management), and many others.
- How do you create an EC2 instance on AWS?
- You can create an EC2 instance through the AWS Management Console, AWS CLI, or Infrastructure as Code tools like Terraform.
- What is AWS S3 used for?
- AWS S3 (Simple Storage Service) is used for scalable object storage. It is commonly used for backup, archiving, and serving static content.
- What is IAM in AWS?
- IAM (Identity and Access Management) is a service that helps you securely control access to AWS resources for users and groups.
- What is a VPC?
- A VPC (Virtual Private Cloud) is a logically isolated section of the AWS cloud where you can launch AWS resources in a virtual network that you define.
- What is the difference between a public and private subnet?
- A public subnet is a subnet that has a route to an internet gateway, allowing the instances within it to communicate with the internet. A private subnet does not have a route to an internet gateway, making it isolated from the internet.
- What is a Load Balancer?
- A load balancer distributes incoming network traffic across multiple servers to ensure no single server becomes overwhelmed, improving application availability and reliability.
- What are the types of Load Balancers in AWS?
- Application Load Balancer (ALB), Network Load Balancer (NLB), and Classic Load Balancer (CLB).
- What is DNS?
- DNS (Domain Name System) is a system that translates human-readable domain names (e.g., www.example.com) into IP addresses that computers use to identify each other on the network.
- What is DevSecOps?
- DevSecOps is the practice of integrating security practices within the DevOps process to ensure that security is a shared responsibility throughout the development lifecycle.
- What are some common security practices in DevOps?
- Code analysis, automated security testing, vulnerability management, access control, and encryption.
- How do you manage secrets in a DevOps environment?
- Using tools like HashiCorp Vault, AWS Secrets Manager, Azure Key Vault, or Kubernetes Secrets.
- What is the principle of least privilege?
- The principle of least privilege is a security concept where users are granted the minimum levels of access – or permissions – needed to perform their job functions.
- How do you ensure data encryption in transit?
- By using TLS/SSL to encrypt data transmitted between clients and servers.
- Why is automation important in DevOps?
- Automation reduces manual effort, minimizes human error, speeds up processes, and ensures consistency and repeatability in tasks such as deployment, testing, and configuration.
- What are some tasks that can be automated in DevOps?
- Code integration, testing, deployment, configuration management, and infrastructure provisioning.
- What is a CI/CD pipeline?
- A CI/CD pipeline is an automated sequence of processes that facilitates the integration and delivery of code changes to production environments, including steps like build, test, and deploy.
- What are some popular automation tools in DevOps?
- Jenkins, GitLab CI, Travis CI, CircleCI, Ansible, and Terraform.
- How do you automate infrastructure provisioning?
- Using Infrastructure as Code (IaC) tools like Terraform, AWS CloudFormation, or Ansible.
- Why are scripting skills important for a DevOps engineer?
- Scripting skills are important for automating tasks, managing configurations, and integrating various tools and processes within the DevOps workflow.
- What are some common scripting languages used in DevOps?
- Bash, Python, Ruby, and PowerShell.
- How do you write a simple Bash script to print “Hello World”?bashCopy code
#!/bin/bash echo "Hello World"
- What is the shebang (
#!/bin/bash
) in a script?- The shebang (
#!/bin/bash
) is a directive used in script files to specify the path to the interpreter that should be used to execute the script.
- The shebang (
- How do you execute a Python script?
python script.py
- What is a relational database?
- A relational database is a type of database that stores data in tables with rows and columns, and the relationships between data are defined by foreign keys.
- What is SQL?
- SQL (Structured Query Language) is a standard programming language used to manage and manipulate relational databases.
- What are some popular relational databases?
- MySQL, PostgreSQL, Oracle, and Microsoft SQL Server.
- What is a NoSQL database?
- A NoSQL database is a type of database that stores data in a format other than relational tables, such as key-value pairs, documents, or graphs.
- What are some popular NoSQL databases?
- MongoDB, Cassandra, Redis, and Couchbase.
- Why is testing important in DevOps?
- Testing ensures that code changes do not introduce bugs or regressions, and helps maintain the quality and reliability of software.
- What are the different types of testing?
- Unit testing, integration testing, functional testing, performance testing, and security testing.
- What is unit testing?
- Unit testing involves testing individual components or functions of the software in isolation to ensure they work as expected.
- What are some popular unit testing frameworks?
- JUnit for Java, pytest for Python, Mocha for JavaScript, and NUnit for .NET.
- What is test automation?
- Test automation involves using software tools to run tests automatically, manage test data, and compare actual outcomes with predicted outcomes.
- What is Agile methodology?
- Agile methodology is an iterative approach to software development that emphasizes flexibility, collaboration, customer feedback, and rapid delivery of small, functional parts of a project.
- What is Scrum?
- Scrum is a framework within Agile methodology that uses fixed-length iterations called sprints, typically 2-4 weeks long, to deliver incremental improvements to the software.
- What are the roles in a Scrum team?
- Product Owner, Scrum Master, and Development Team.
- What is a sprint?
- A sprint is a fixed time period, usually 2-4 weeks, during which a Scrum team works to complete a set of predefined tasks or goals.
- What is a daily stand-up meeting?
- A daily stand-up meeting is a short meeting, usually around 15 minutes, where team members share their progress, plans, and any blockers they are facing.
- Why is communication important in DevOps?
- Effective communication ensures that all team members are aligned, issues are quickly addressed, and collaboration is facilitated across development, operations, and other stakeholders.
- What are some tools used for communication and collaboration in DevOps?
- Slack, Microsoft Teams, Zoom, Jira, Confluence, and GitHub.
- What is a post-mortem meeting?
- A post-mortem meeting is held after an incident or project completion to analyze what went well, what went wrong, and how processes can be improved in the future.
- How do you handle conflicts in a DevOps team?
- By promoting open communication, understanding different perspectives, focusing on the problem rather than personal differences, and finding a collaborative solution.
- What is a blameless culture?
- A blameless culture focuses on learning and improvement rather than assigning blame when things go wrong. It encourages transparency and accountability without fear of punishment.
- What is scalability in the context of DevOps?
- Scalability is the ability of a system to handle increased load by adding resources, either by scaling up (vertical scaling) or scaling out (horizontal scaling).
- What is vertical scaling?
- Vertical scaling involves adding more power (CPU, RAM) to an existing server.
- What is horizontal scaling?
- Horizontal scaling involves adding more servers to handle the load.
- What are some common performance metrics to monitor?
- CPU usage, memory usage, disk I/O, network latency, and response time.
- What is a load test?
- A load test measures the system’s performance under a specific expected load to determine how it behaves under normal and peak conditions.
- What is compliance in the context of DevOps?
- Compliance ensures that the systems and processes adhere to regulatory requirements, industry standards, and organizational policies.
- What are some common compliance frameworks?
- GDPR, HIPAA, PCI-DSS, SOC 2, and ISO/IEC 27001.
- How do you ensure compliance in a DevOps pipeline?
- By incorporating automated compliance checks, using compliance-as-code tools, and regularly auditing and reviewing processes.
- What is governance in DevOps?
- Governance involves establishing policies, processes, and controls to ensure that IT resources are effectively and efficiently managed to meet organizational goals.
- What is audit logging?
- Audit logging involves recording all significant actions and events within a system to provide a trail that can be used to track changes and ensure accountability.
- What is incident management?
- Incident management is the process of identifying, analyzing, and responding to incidents to minimize their impact and restore normal operations as quickly as possible.
- What are the stages of incident management?
- Identification, categorization, prioritization, response, resolution, and closure.
- What is an SLA (Service Level Agreement)?
- An SLA is a contract between a service provider and a customer that specifies the expected level of service, including metrics like uptime, response time, and resolution time.
- What is an RTO (Recovery Time Objective)?
- RTO is the maximum acceptable amount of time that a system can be offline after an incident before operations must be restored.
- What is an RPO (Recovery Point Objective)?
- RPO is the maximum acceptable amount of data loss measured in time. It determines how often data backups should occur.
- What is a microservice?
- A microservice is an architectural style that structures an application as a collection of small, loosely coupled, independently deployable services.
- What are the benefits of microservices?
- Scalability, flexibility, resilience, and faster time to market.
- What is a service mesh?
- A service mesh is a dedicated infrastructure layer for handling service-to-service communication, providing features like load balancing, service discovery, and security.
- What is Kubernetes?
- Kubernetes is an open-source container orchestration platform for automating the deployment, scaling, and management of containerized applications.
- What is a Helm chart?
- A Helm chart is a package manager for Kubernetes, providing a way to define, install, and upgrade even the most complex Kubernetes applications.
- What is a blue-green deployment?
- Blue-green deployment is a technique that reduces downtime and risk by running two identical production environments (blue and green) and switching traffic between them during a deployment.
- What is canary deployment?
- Canary deployment is a technique where a new version of a service is gradually rolled out to a small subset of users before being deployed to the entire user base.
- What is chaos engineering?
- Chaos engineering involves intentionally introducing failures into a system to test its resilience and understand how it behaves under unexpected conditions.
- What is a build artifact?
- A build artifact is a file or set of files produced by the build process, which can include compiled code, libraries, executables, or configuration files.
- What is an API Gateway? – An API Gateway is a server that acts as an API front-end, handling requests, routing them to appropriate backend services, and providing additional functionalities like load balancing, security, and rate limiting.
Posted inDevOps Engineering Interviews
Top DevOps Engineer Interview Questions and Answers for 2024
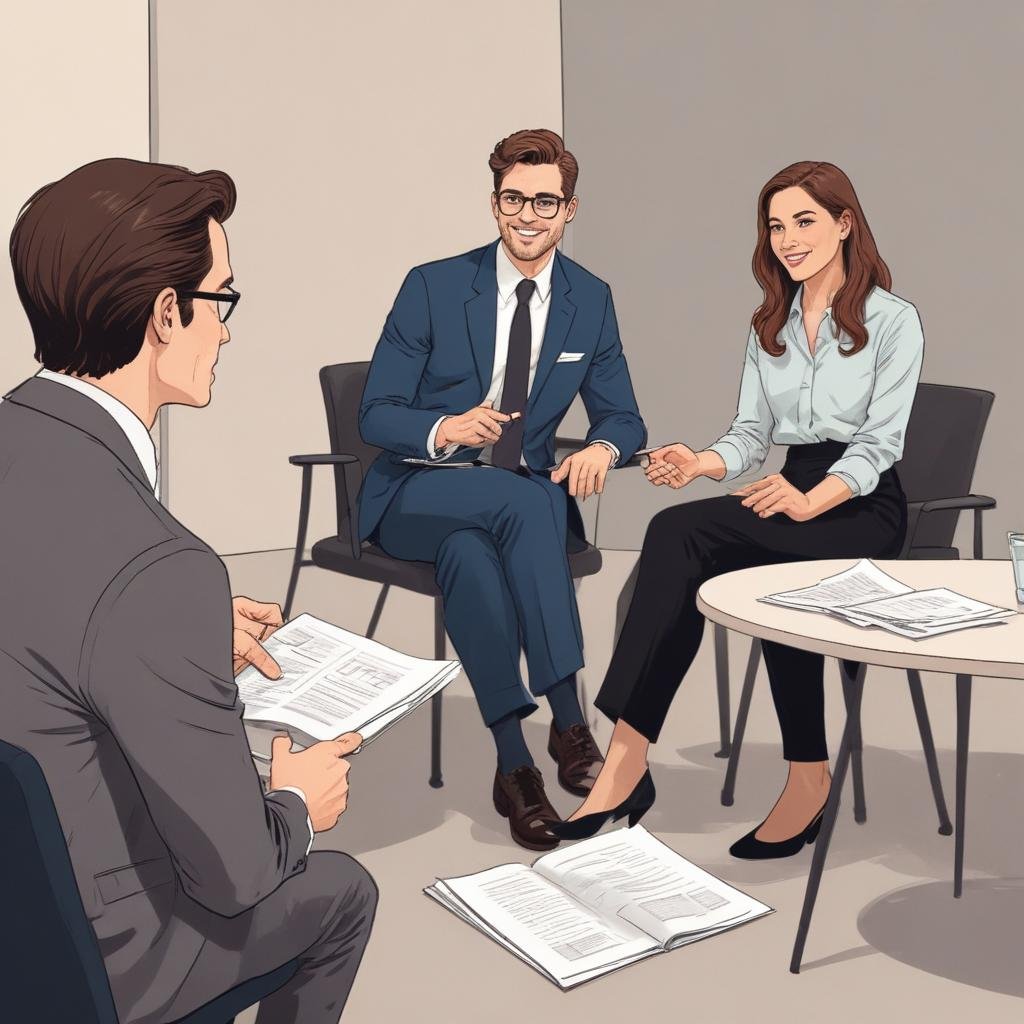
Reading Time: 9 minutes